<?php class student{ public $name; public $lastname; public $bilet; public $group; public function __construct(string $name,string $lastname,string $group) { $this->name = $name; $this->lastname = $lastname; $this->group = $group; $this->bilet = rand(0,999); } public function displayStudentInfo(){ $bilet = $this->bilet; if ($bilet) { echo 'Группа:'. $this->group .'<br/>'; echo 'Студенческий билет: '.$bilet.'<br/>'; } echo '<ul style="padding-left:15px;">'; echo 'Имя:'. $this->name .'<br/>'; echo 'Фамилия:'. $this->lastname .'<br/>'; echo '</ul>'; } } $students = [ new Student("Вася","Пупкин","ЭУ-220"), new Student("Петя","Васильев","ЭУ-220"), new Student("Даня","Коста","ЭУ-220") ]; for ($j = 0; $j < count($students) - 1; $j++){ for ($i = 0; $i < count($students) - $j - 1; $i++){ if ($students[$i]->bilet > $students[$i + 1]->bilet){ $tmp_var = $students[$i + 1]; $students[$i + 1] = $students[$i]; $students[$i] = $tmp_var; } } } foreach($students as $student){ echo $student->displayStudentInfo(); } ?>
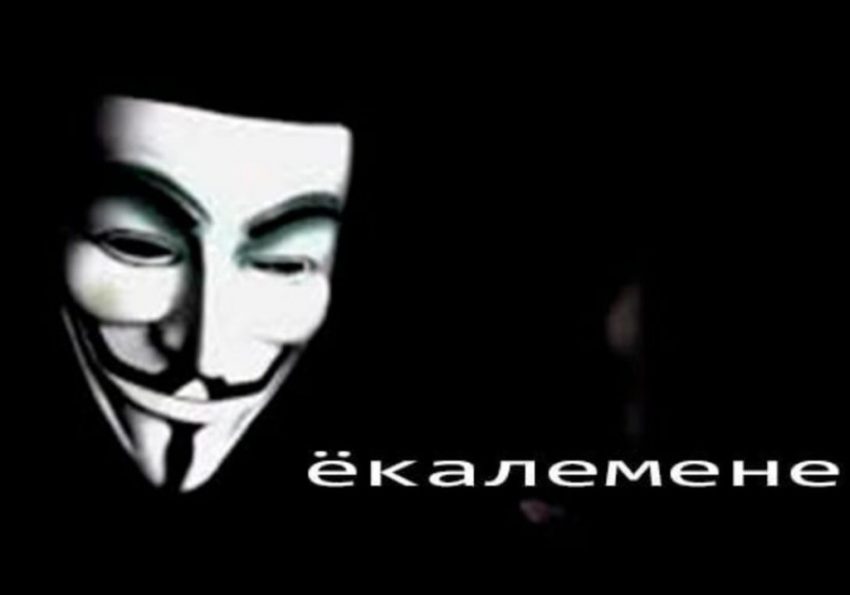